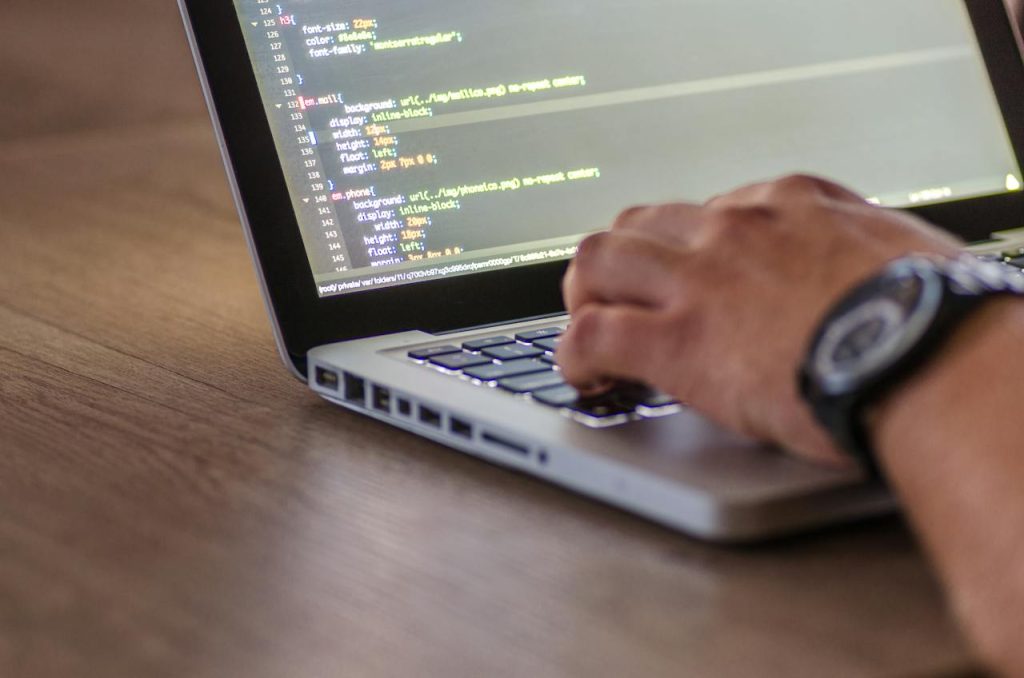
Image source link : https://www.pexels.com/pl-pl/zdjecie/osoba-kodujaca-w-laptopie-574071/
In the vast universe of web development, there might be moments when you find yourself feeling like a tiny, lost astronaut without a map.
For many developers, one of those moments is when they first consider integrating Node.js with modern frontend frameworks.
It’s not just about slapping together a server-side environment with a flashy interface; it’s about creating a seamless conduit for high-performance, real-time user experiences that can make or break your dynamic web application.
Before you can start offering Node.js development services, you must master both the Node.js and frameworks like React, Angular, or Vue.
How do you synchronize the backend beat with the frontend tune without stepping on any toes?
Read one, and you’ll find yourself orchestrating a web symphony that not only performs flawlessly but also scales elegantly with your users’ demands.
Key Takeaways
- Node.js is a powerful JavaScript runtime environment for building fast and scalable network applications.
- Frontend frameworks like React, Angular, and Vue.js can be used to create dynamic and sophisticated single-page applications.
- Setting up the development environment involves installing Node.js, choosing a code editor, and utilizing package managers like NPM or Yarn.
- Integrating the Node.js backend with frontend frameworks requires creating RESTful API endpoints, handling CORS for secure communication, implementing JWT for authentication, and optimizing JSON payloads for improved loading times.
Node.js Basics
Understanding the core concepts of Node.js is crucial to integrate it with various frontend frameworks.
Node.js is a powerful, open-source, cross-platform JavaScript runtime environment that executes JavaScript code outside a web browser. You’ll find it especially useful for building fast, scalable network applications.
Node.js utilizes an event-driven, non-blocking I/O model, which makes it lightweight and efficient. This model is ideal for data-intensive real-time applications that run across distributed devices.
Moreover, Node.js operates on a single-threaded event loop, contrary to traditional multi-threaded server models. It means you can handle numerous connections concurrently without incurring the cost of thread context switching.
You’ll interact with Node.js through its package manager, NPM, which is the largest ecosystem of open-source libraries in the world.
When you’re working with frontend frameworks like React, Angular, or Vue.js, NPM allows you to manage dependencies and install packages that can extend the functionality of your applications.
Exploring Frontend Frameworks
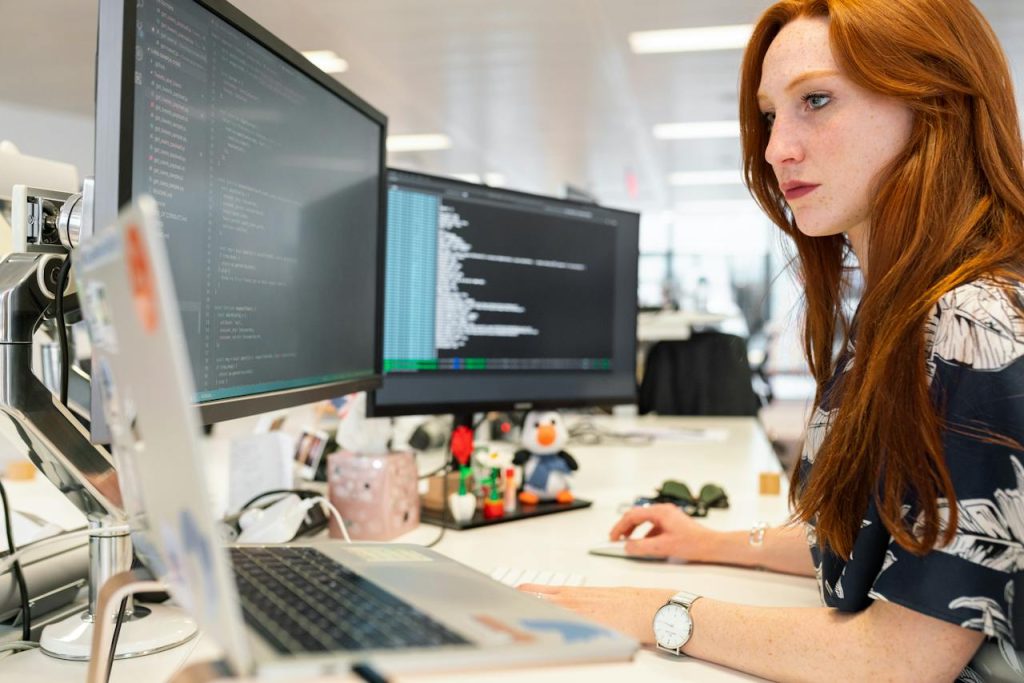
Image source: https://www.pexels.com/pl-pl/zdjecie/kobieta-koduje-na-komputerze-3861958/
Tools like React, Angular, and Vue.js are designed to simplify the development process, offering robust features and streamlined workflows that cater to the demands of modern web application development.
Each framework comes with its own set of conventions, best practices, and community support, which are pivotal in crafting scalable and maintainable web applications.
Consider the following aspects of frontend frameworks:
- React: A JavaScript library for building user interfaces, known for its virtual DOM and component-based architecture.
- Angular: A platform and framework for building single-page client applications using HTML and TypeScript.
- Vue.js: A progressive framework that’s adaptable and approachable, ideal for building sophisticated single-page applications.
Your choice of a frontend framework should align with project requirements, team expertise, and long-term maintainability. Each provides a structured approach to organizing code, managing state, and optimizing user experience, ensuring your web applications are both performant and easy to use.
Setting Up the Development Environment
Before you can harness the power of your chosen frontend framework, you’ll need to establish a development environment tailored to Node.js and your specific framework. This setup is crucial for efficient development and testing of your web applications.
Firstly, you should install Node.js, which includes NPM (Node Package Manager). NPM is essential for managing packages that your project depends on. Ensure you download the LTS (Long Term Support) version for stability.
Next, select a code editor suited for JavaScript development, such as Visual Studio Code or Atom, which offer features like syntax highlighting and code completion.
Here’s a quick guide for setting up the development environment:
Requirement | Suggestion |
Node.js | Install the latest LTS version from the official Node.js website. |
Code Editor | Choose Visual Studio Code or Atom for their extensive support for JavaScript and Node.js. |
Package Manager | Utilize NPM or Yarn to manage your project dependencies efficiently. |
Once your development tools are installed, initialize a new Node.js project by running `npm init` in your project directory. This command creates a `package.json` file, which is the blueprint for your project’s dependencies and scripts.
Building the Node.js Backend
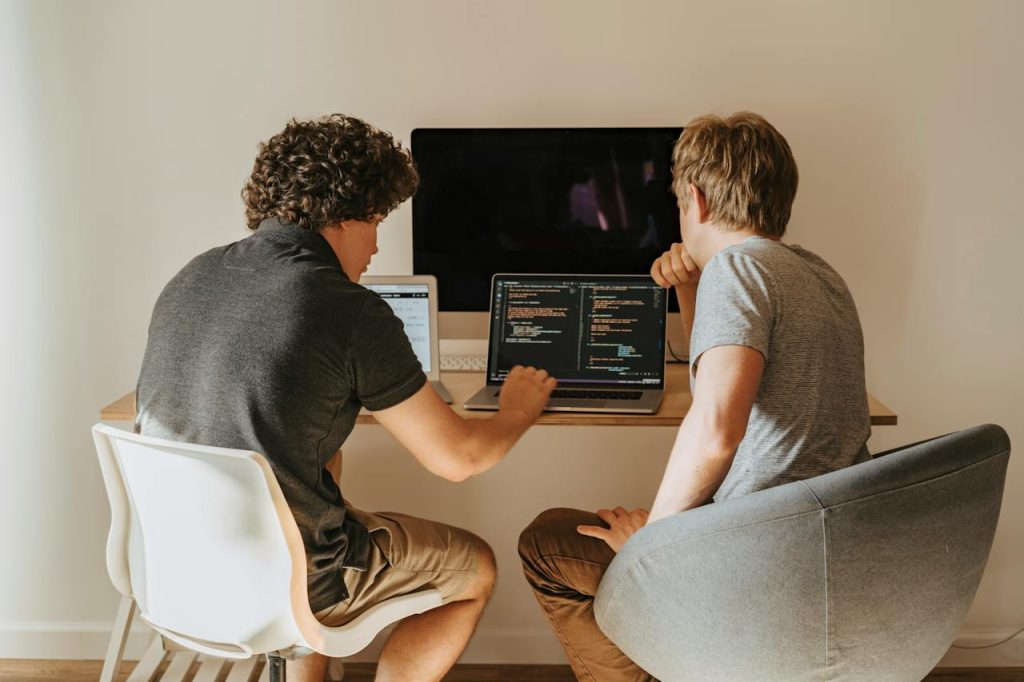
Image source: https://www.pexels.com/pl-pl/zdjecie/mezczyzna-ludzie-laptop-mezczyzni-4974920/
Now, it’s time to focus on constructing the Node.js backend that will power your application’s server-side logic. You’ll harness Node.js’s non-blocking I/O model to build a scalable and performant system capable of handling numerous concurrent connections without breaking a sweat.
Delve into the following facets to ensure your backend’s robustness and responsiveness:
- Initialization of a Node.js Project: Start by using `npm init` to create your `package.json` file, which will manage your project’s dependencies and scripts.
- Express Framework Setup: Leverage Express, a minimal and flexible Node.js web application framework, to handle HTTP requests with ease.
- Database Integration: Choose a database that fits your needs, be it a NoSQL option like MongoDB or a relational database like PostgreSQL, and integrate it using appropriate Node.js drivers or ORMs.
- RESTful API Development: Design and implement RESTful routes that enable CRUD operations, ensuring seamless data flow between your frontend and backend.
- Middleware Utilization: Incorporate middleware for tasks such as parsing JSON payloads, handling cookies, and securing your endpoints through authentication and authorization mechanisms.
Integrating Backend With Frontend
Once you’ve established a solid Node.js backend, integrate it with your frontend to create a cohesive user experience.
This integration is pivotal because it enables the two layers of your application to communicate effectively and deliver dynamic content to users.
To achieve this, you’ll need to:
- Create RESTful API endpoints: Your Node.js server should expose a set of API endpoints that the frontend can consume to perform actions and retrieve data.
- Handle CORS (Cross-Origin Resource Sharing): Ensure your backend is configured to accept requests from the domain where your frontend is hosted to avoid security issues.
- Implement JSON Web Tokens (JWT) for secure authentication: Use tokens to manage user sessions and secure API endpoints, allowing only authenticated users to access certain resources.
- Optimize data transfer: Structure your JSON payloads efficiently to reduce latency and improve loading times for your users.
- Utilize WebSockets for real-time communication: For features that require instant updates, like chat applications or live feeds, incorporate WebSockets to facilitate a two-way interactive communication session.
Final Thoughts
You’ve now mastered the art of combining Node.js with leading frontend frameworks to craft dynamic web applications.
Remember, ensure your development environment is precise, build a robust Node.js backend, and seamlessly integrate it with your chosen frontend.
This synergy is crucial for responsive, real-time user experiences.
Embrace each challenge as an opportunity to innovate and excel in the web development arena!
Frequently Asked Questions
How Does Server-Side Rendering With Node.Js Affect SEO?
Server-side rendering with Node.js improves SEO as search engines can index your content more easily than with client-side rendering, which often requires additional time and processing to become searchable.
Can Node.Js Be Integrated With Frontend Frameworks to Create Progressive Web Apps?
Yes, you can integrate Node.js with frontend frameworks to build PWAs. Best practices include using service workers, implementing responsive design, and ensuring offline functionality for a seamless user experience.
How Do You Handle Authentication and Authorization Securely When Integrating Node.Js With Frontend Frameworks?
To handle authentication and authorization securely, you’ll want to implement HTTPS, use tokens like JWT for sessions, and ensure password hashing. Always validate user input and manage permissions on the server side.
What Are the Performance Implications of Using Node.Js With Large-Scale Frontend Frameworks?
You’ll experience increased load times and potential bottlenecks with Node.js in large-scale projects. Optimize by profiling performance, streamlining code, and implementing efficient caching and load balancing strategies.
How Can You Facilitate Real-Time Data Communication Between Node.Js and Frontend Frameworks for Features Like Chat Applications or Live Updates?
You’ll want to use WebSockets or libraries like Socket.IO for real-time communication, ensuring efficient data exchange and low latency for features like chat or live updates in your applications.